Description
A ruby library/gem for interacting with .docx files. currently capabilities include reading paragraphs/bookmarks, inserting text at bookmarks, reading tables/rows/columns/cells and saving the document.
docx alternatives and similar gems
Based on the "Spreadsheets and Documents" category.
Alternatively, view docx alternatives based on common mentions on social networks and blogs.
-
AXLSX
xlsx generation with charts, images, automated column width, customizable styles and full schema validation. Axlsx excels at helping you generate beautiful Office Open XML Spreadsheet documents without having to understand the entire ECMA specification. Check out the README for some examples of how easy it is. Best of all, you can validate your xlsx file before serialization so you know for sure that anything generated is going to load on your client's machine. -
Spreadsheet Architect
Spreadsheet Architect is a library that allows you to create XLSX, ODS, or CSV spreadsheets super easily from ActiveRecord relations, plain Ruby objects, or tabular data. -
Xsv .xlsx reader
High performance, lightweight .xlsx parser for Ruby that provides nothing a CSV parser wouldn't
WorkOS - The modern identity platform for B2B SaaS
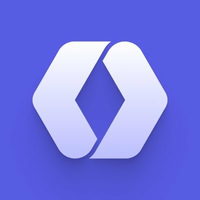
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of docx or a related project?
Popular Comparisons
README
docx
A ruby library/gem for interacting with .docx
files. currently capabilities include reading paragraphs/bookmarks, inserting text at bookmarks, reading tables/rows/columns/cells and saving the document.
Usage
Prerequisites
- Ruby 2.5 or later
Install
Add the following line to your application's Gemfile:
gem 'docx'
And then execute:
bundle install
Or install it yourself as:
gem install docx
Reading
require 'docx'
# Create a Docx::Document object for our existing docx file
doc = Docx::Document.open('example.docx')
# Retrieve and display paragraphs
doc.paragraphs.each do |p|
puts p
end
# Retrieve and display bookmarks, returned as hash with bookmark names as keys and objects as values
doc.bookmarks.each_pair do |bookmark_name, bookmark_object|
puts bookmark_name
end
Don't have a local file but a buffer? Docx handles those to:
require 'docx'
# Create a Docx::Document object from a remote file
doc = Docx::Document.open(buffer)
# Everything about reading is the same as shown above
Rendering html
require 'docx'
# Retrieve and display paragraphs as html
doc = Docx::Document.open('example.docx')
doc.paragraphs.each do |p|
puts p.to_html
end
Reading tables
require 'docx'
# Create a Docx::Document object for our existing docx file
doc = Docx::Document.open('tables.docx')
first_table = doc.tables[0]
puts first_table.row_count
puts first_table.column_count
puts first_table.rows[0].cells[0].text
puts first_table.columns[0].cells[0].text
# Iterate through tables
doc.tables.each do |table|
table.rows.each do |row| # Row-based iteration
row.cells.each do |cell|
puts cell.text
end
end
table.columns.each do |column| # Column-based iteration
column.cells.each do |cell|
puts cell.text
end
end
end
Writing
require 'docx'
# Create a Docx::Document object for our existing docx file
doc = Docx::Document.open('example.docx')
# Insert a single line of text after one of our bookmarks
doc.bookmarks['example_bookmark'].insert_text_after("Hello world.")
# Insert multiple lines of text at our bookmark
doc.bookmarks['example_bookmark_2'].insert_multiple_lines_after(['Hello', 'World', 'foo'])
# Remove paragraphs
doc.paragraphs.each do |p|
p.remove! if p.to_s =~ /TODO/
end
# Substitute text, preserving formatting
doc.paragraphs.each do |p|
p.each_text_run do |tr|
tr.substitute('_placeholder_', 'replacement value')
end
end
# Save document to specified path
doc.save('example-edited.docx')
Advanced
require 'docx'
d = Docx::Document.open('example.docx')
# The Nokogiri::XML::Node on which an element is based can be accessed using #node
d.paragraphs.each do |p|
puts p.node.inspect
end
# The #xpath and #at_xpath methods are delegated to the node from the element, saving a step
p_element = d.paragraphs.first
p_children = p_element.xpath("//child::*") # selects all children
p_child = p_element.at_xpath("//child::*") # selects first child
Development
todo
- Calculate element formatting based on values present in element properties as well as properties inherited from parents
- Default formatting of inserted elements to inherited values
- Implement formattable elements.
- Implement styles.
- Easier multi-line text insertion at a single bookmark (inserting paragraph nodes after the one containing the bookmark)