Description
Shrine implements a plugin system analogous to Roda’s and Sequel’s. It has a small core which provides only the essential functionality, while other features come as plugins which can be loaded when needed. Shrine ships with over 25 plugins, which together provide a great arsenal of features.
This design makes Shrine extremely versatile. File uploads are very delicate, and need to be handled differently depending on what types of files are being uploaded, whether there is processing or not, what storage is used etc. Instead of having an opinion on how you want to do your upload, Shrine allows you to build an uploading flow that suits your needs.
Shrine alternatives and similar gems
Based on the "File Upload" category.
Alternatively, view Shrine alternatives based on common mentions on social networks and blogs.
-
DragonFly
A Ruby gem for on-the-fly processing - suitable for image uploading in Rails, Sinatra and much more!
InfluxDB - Power Real-Time Data Analytics at Scale
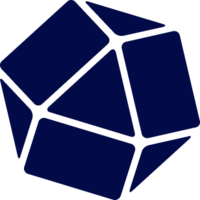
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Shrine or a related project?
Popular Comparisons
README
Shrine
Shrine is a toolkit for handling file attachments in Ruby applications. Some highlights:
- Modular design – the plugin system allows you to load only the functionality you need
- Memory friendly – streaming uploads and downloads make it work great with large files
- Cloud storage – store files on disk, AWS S3, Google Cloud, Cloudinary and others
- Persistence integrations – works with Sequel, ActiveRecord, ROM, Hanami and Mongoid and others
- Flexible processing – generate thumbnails eagerly or on-the-fly using ImageMagick or libvips
- Metadata validation – validate files based on extracted metadata
- Direct uploads – upload asynchronously to your app or to the cloud using Uppy
- Resumable uploads – make large file uploads resumable on S3 or tus
- Background jobs – built-in support for background processing that supports any backgrounding library
If you're curious how it compares to other file attachment libraries, see the Advantages of Shrine. Otherwise, follow along with the Getting Started guide.
Links
Resource | URL |
---|---|
Website & Documentation | shrinerb.com |
Demo code | Roda / Rails |
Wiki | github.com/shrinerb/shrine/wiki |
Discussion forum | github.com/shrinerb/shrine/discussions |
Alternate Discussion forum | discourse.shrinerb.com |
Setup
Run:
bundle add shrine
Then add config/initializers/shrine.rb
which sets up the storage and loads
ORM integration:
require "shrine"
require "shrine/storage/file_system"
Shrine.storages = {
cache: Shrine::Storage::FileSystem.new("public", prefix: "uploads/cache"), # temporary
store: Shrine::Storage::FileSystem.new("public", prefix: "uploads"), # permanent
}
Shrine.plugin :activerecord # loads Active Record integration
Shrine.plugin :cached_attachment_data # enables retaining cached file across form redisplays
Shrine.plugin :restore_cached_data # extracts metadata for assigned cached files
Next, add the <name>_data
column to the table you want to attach files to. For
an "image" attachment on a photos
table this would be an image_data
column:
$ rails generate migration add_image_data_to_photos image_data:text # or :jsonb
If using jsonb
consider adding a gin index for fast key-value pair searchability within image_data
.
Now create an uploader class (which you can put in app/uploaders
) and
register the attachment on your model:
class ImageUploader < Shrine
# plugins and uploading logic
end
class Photo < ActiveRecord::Base
include ImageUploader::Attachment(:image) # adds an `image` virtual attribute
end
In our views let's now add form fields for our attachment attribute that will allow users to upload files:
<%= form_for @photo do |f| %>
<%= f.hidden_field :image, value: @photo.cached_image_data, id: nil %>
<%= f.file_field :image %>
<%= f.submit %>
<% end %>
When the form is submitted, in your controller you can assign the file from request params to the attachment attribute on the model:
class PhotosController < ApplicationController
def create
Photo.create(photo_params) # attaches the uploaded file
# ...
end
private
def photo_params
params.require(:photo).permit(:image)
end
end
Once a file is uploaded and attached to the record, you can retrieve the file URL and display it on the page:
<%= image_tag @photo.image_url %>
See the Getting Started guide for further documentation.
Inspiration
Shrine was heavily inspired by Refile and Roda. From Refile it borrows the idea of "backends" (here named "storages"), attachment interface, and direct uploads. From Roda it borrows the implementation of an extensible plugin system.
Similar libraries
- Paperclip
- CarrierWave
- Dragonfly
- Refile
- Active Storage
Contributing
Please refer to the contributing page.
Code of Conduct
Everyone interacting in the Shrine project’s codebases, issue trackers, and mailing lists is expected to follow the Shrine code of conduct.
License
The gem is available as open source under the terms of the MIT License.
*Note that all licence references and agreements mentioned in the Shrine README section above
are relevant to that project's source code only.