Description
All of sudden, one thing hit me very. Ruby is a cool dev friendly dynamic language. Even though its cool, there we lot of occasions where we need to validate structure of the data like payload structure, arguments like options(hash) which is common in methods etc. So came up with below tiny library :)
Simple DSL for datatype validation for basic data types.
Inspired from GRPC DSL.
The purpose of this library is strictly not for application validations like email, pattern matching. This is purely for datatype matching. Since ruby is dynamic language, usecases where strict datatypes should be enforced this library will be helpful.
data_type_validator alternatives and similar gems
Based on the "Web Frameworks" category.
Alternatively, view data_type_validator alternatives based on common mentions on social networks and blogs.
-
ReactOnRails
Integration of React + Webpack + Rails + rails/webpacker including server-side rendering of React, enabling a better developer experience and faster client performance. -
Glimmer DSL for Opal
DISCONTINUED. Glimmer DSL for Opal (Pure-Ruby Web GUI and Auto-Webifier of Desktop Apps)
InfluxDB - Power Real-Time Data Analytics at Scale
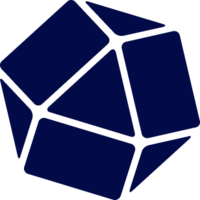
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of data_type_validator or a related project?
README
data_type_validator
Simple DSL for datatype validation for basic data types. Inspired from GRPC DSL. The purpose of this library is strictly not for application validations like email, pattern matching. This is purely for datatype matching. Since ruby is dynamic language, usecases where strict datatypes should be enforced this library will be helpful.
installation
gem install data_type_validator
Usage
Structure Definition
class Custom < DataTypeValidator::CustomContainer
attribute_def :string_attr, :string
attribute_def :integer_attr, :integer
attribute_def :float_attr, :float
attribute_def :boolean_attr, :boolean
end
Validations
return true if valid
throws exception DataTypeValidator::Errors::InvalidTypeDefinitions if invalid
Custom.validate({ string_attr: "1", integer_attr: 1, float_attr: 1.21, boolean_attr: true })
attribute_def
> attribute_def :name(attribute key), :type(data_type)
> valid types are :string, :integer, :float, :boolean, DataTypeValidator::CustomContainer
Array attribute Definition
class Custom < DataTypeValidator::CustomContainer
array_attribute_def :array_attr, :string
end
invocation
Custom.validate({ array_attr: ["1", "2"] })
Nested hash attribute definition
class NestedCustom < DataTypeValidator::CustomContainer
attribute_def :string_attr, :string
end
class Custom < DataTypeValidator::CustomContainer
attribute_def :nested_attr, NestedCustom.type_alias
array_attribute_def :array_attr, :string
end
invocation
Custom.validate({ array_attr: ["1", "2"], nested_attr: { string_attr: "1" } })
NOTES
- Does not support inheritance. always inherit from DataTypeValidator::CustomContainer.
- The purpose of this library is only type validation. We intended to avoid multilevel inheritance, other complex patterns.
- We follow GRPC DSL. Neat and clean.